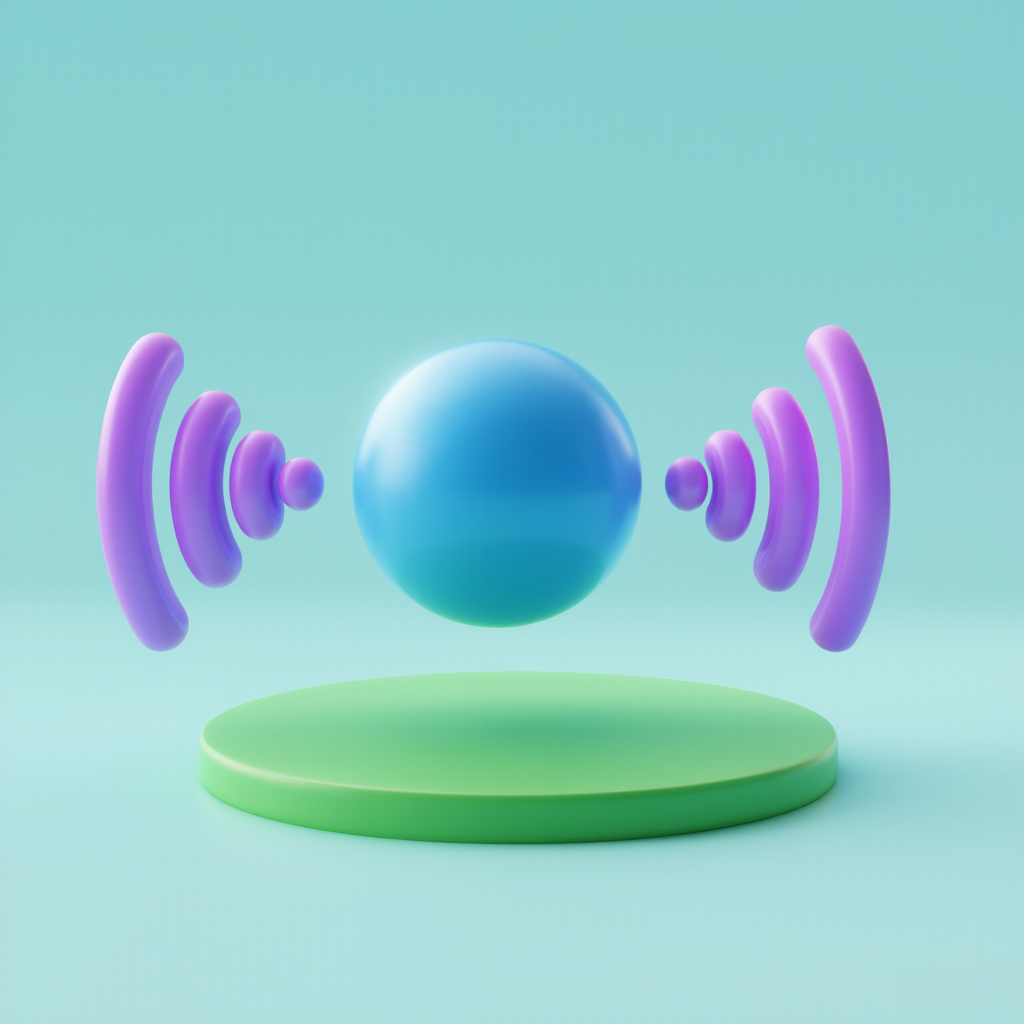
A Unity script that plays an audio file when an object collides with a specific plane.
To use this script:
- Create a new script in Unity and name it “CollisionAudioPlayer”
- Copy the code into the script:
C#
using UnityEngine;
public class CollisionAudioPlayer : MonoBehaviour
{
[Header("Audio Settings")]
[SerializeField] private AudioClip collisionSound;
[SerializeField] private float volume = 1f;
[SerializeField] private bool playOnce = false;
[Header("Collision Settings")]
[SerializeField] private string targetTag = "Ground";
[SerializeField] private float minCollisionForce = 0.1f;
private AudioSource audioSource;
private bool hasPlayed = false;
private void Start()
{
// Add AudioSource component if it doesn't exist
audioSource = GetComponent<AudioSource>();
if (audioSource == null)
{
audioSource = gameObject.AddComponent<AudioSource>();
}
// Configure AudioSource
audioSource.playOnAwake = false;
audioSource.clip = collisionSound;
audioSource.volume = volume;
}
private void OnCollisionEnter(Collision collision)
{
// Check if we should play only once and have already played
if (playOnce && hasPlayed)
return;
// Check if we collided with the target object
if (collision.gameObject.CompareTag(targetTag))
{
// Check if collision force is strong enough
if (collision.relativeVelocity.magnitude >= minCollisionForce)
{
audioSource.Play();
hasPlayed = true;
}
}
}
// Method to reset the play state if needed
public void ResetPlayState()
{
hasPlayed = false;
}
}
3. Attach the script to the object that you want to detect collisions from
4. In the Unity Inspector, you’ll need to:
- Assign an AudioClip to the “Collision Sound” field
- Set the “Target Tag” to match the tag of your plane (default is “Ground”)
- Adjust the volume if needed
- Set “Play Once” if you want the sound to play only on the first collision
- Adjust “Min Collision Force” to set how strong the collision needs to be to trigger the sound
Make sure your plane has:
- A Collider component (Box Collider, Mesh Collider, etc.)
- The correct tag that matches what you set in the script
The script includes features like:
- Volume control
- Option to play sound only once
- Minimum collision force threshold
- Method to reset the play state
- Automatic AudioSource component addition
You should attach the script to the moving object that will collide with the plane, not to the plane itself. Here’s why:
- If attached to the moving object:
- The script will trigger when that object hits any plane/ground with the correct tag
- Good for things like:
- Footstep sounds for a player character
- Impact sounds for thrown objects
- Landing sounds for jumping objects
- If you attached it to the plane:
- It would play for any object that hits it
- You might get unwanted sounds from other objects hitting the plane
- Could be overwhelming if multiple objects hit at once
Here’s a practical example:
CopyScene Setup:
├── Player (or Ball, or any moving object)
│ ├── CollisionAudioPlayer script
│ ├── Rigidbody
│ └── Collider (Sphere, Box, etc.)
│
└── Ground/Plane
├── Tag: "Ground"
├── Collider
└── Is Trigger: false
Remember to:
- Make sure your moving object has a Rigidbody component
- Tag your plane/ground with the tag you specified in the script (default “Ground”)
- Add your sound file to the “Collision Sound” field in the script’s inspector
Leave a Reply